#
A modern C++-20 unit-testing framework.
Example#
Writing tests is as simple as writing modern, standard C++-20:
#include <clean-test/clean-test.h>
constexpr auto sum(auto... vs) { return (0 + ... + vs); }
namespace ct = clean_test;
using namespace ct::literals;
auto const suite = ct::Suite{"sum", [] {
"0"_test = [] { ct::expect(sum() == 0_i); };
"3"_test = [] { ct::expect(sum(1, 2) == 1 + 2_i); };
"A"_test = [] { ct::expect(sum(-1) < 0_i and sum(+1) > 0_i); };
}};
Key Features#
Although many unit-testing frameworks have been released to date, none combines the following qualities:
Macro Free#
Macros come with many drawbacks: lack of scoping, difficulty to evolve, debug or understand (just to name a few). This often adds up to macro magic which is hardly accessible or discoverable - even for experienced developers.
As a consequence macros are often discouraged in code bases. In contrast to this most unit-testing frameworks heavily rely on macros, particularly for expressing assertions.
Clean Test does not need any macros, but leverages modern, standard C++ instead.
The resulting expression introspection is highly superior
(constexpr
, supports short circuiting).
The few notions of the framework are very easy to discover for any C++ developer.
Parallel#
Modern hardware comes with multi-CPU architectures and many C++-programs make use of these. However, the existing frameworks can only execute the corresponding unit-tests sequentially.
Clean Test on the other hand executes any number of tests simultaneously and can fully benefit from modern hardware. This helps to execute tests more quickly and to identify issues with thread-safety already during testing.
The assertions of Clean Test are thread-safe i.e. simultaneous failures of one assertion can be detected.
This thread safety particularly applies to parallel tests (e.g. based on asio
or some thread pool).
Production Ready#
Clean Test provides all the utilities expected from a unit-testing framework: It has no dependencies and as a CMake library is very easy to build and integrate.
The flexible runtime configuration is particularly useful during development. Many details can be specified dynamically e.g. test selection, execution context as well as output control.
Moreover Clean Test can also be used with any continuous integration tool. With the support of JUnit XML-reports (the most common format) any CI-engine can display error details and statistics. Clean Test guarantees valid UTF-8 output, thus allowing for assertions with arbitrary byte-sequences. The necessary escaping is done automatically, if necessary.
Video#
Details about Clean Test were presented at Meeting C++ 2022 in Berlin:
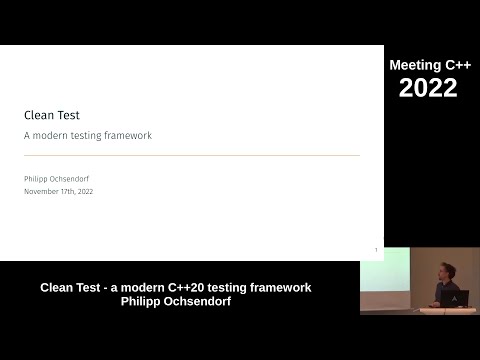